A Random Walker
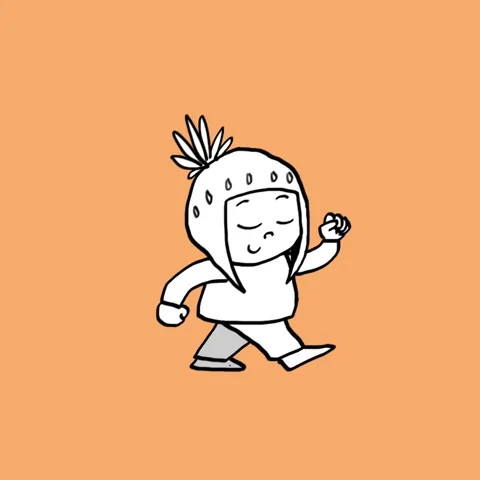
It was a rainy Saturday night when I discovered the incredible YouTube channel The Coding Train by Daniel Shiffman. As I devoured several videos in a row, I felt an excitement for programming that I hadn't experienced in a long time.
In one video, he mentioned his book, The Nature of Code. The name piqued my curiosity; how could nature and code be together? So, I started reading it.
The Nature of Code
The purpose of this book is simple: how to simulate physical phenomena with code.
My sad attempt to create a bee in a green field (Source Code)
From what I understand, the idea behind The Nature of Code (NOC) is to break down the natural world into smaller parts and transform them into code using JavaScript.
The book is packed with physical and mathematical concepts, which intimidated me since I hated these subjects in school. However, all the concepts are presented visually through the p5.js library.
How amazing p5.js is; it’s great for creating art, allowing you to draw through code. It’s simple, fast, and can be run directly in the browser through the editor. All the code in the book uses p5.js.
The base code for p5.js is:
function setup() { createCanvas(400, 400); } function draw() { background(220); }
The setup function is executed only once when the program starts; in this case, it creates a 400x400 pixel canvas.
The draw function runs everything inside it in a loop and is where we draw on the canvas created by the setup. I said, it's simple.
Finally, we arrive at Chapter 0: Randomness.
Randomness
Randomness in computing is a lie. In reality, computers generate pseudo-random numbers, meaning that over time, the numbers start to repeat. This period is so long that usually the generated numbers are sufficient for most things.
With this, we are introduced to the concept of the Walker. A point on the canvas that moves randomly:
A simple Walker (Source Code)
How can something so simple be so cool?
It's just a little dot that, in each loop of draw, chooses a direction to go and then starts again. I found this so amazing that from this point on, I couldn't stop reading NOC.
The problem, according to what I understood from the author, is that pure randomness is not a good design for creating an organic simulation; we need some way to produce random numbers where some results are more likely than others. That's where the Gaussian distribution comes in.
Gaussian Distribution
The Gaussian distribution is a way where numbers cluster around a mean value. And through something called standard deviation, the randomness can accumulate near this mean or not.
We can see this in this drawing:
Points that appear following the Gaussian distribution (Source Code)
If you slide the slider to the right, the points become more random, but to the left, the points tend to concentrate in the middle.
Thus, the Walker using the Gaussian distribution looks like this:
The Walker using Gaussian distribution (Source Code)
However, there is a problem with the Walker that uses pure randomness and Gaussian distribution: they return multiple times to already visited positions (oversampling). The NOC presents a solution: Lévy Flight.
Lévy Flight
I found this name really cool; it would make a good movie title, I think.
Anyway, one way to avoid oversampling is to make our Walker jump large distances occasionally, reducing the number of times the Walker passes through the same place.
A simple way to implement Lévy Flight is: the larger the step, the smaller the chance of being chosen, and the smaller the step, the greater the chance.
So it looks like this:
A Walker with a simple implementation of Lévy Flight (Source Code)
The problem is that Lévy Flight are not so smooth; that's where Perlin Noise comes in.
Perlin Noise
Since randomness isn't that natural, we need to generate random numbers in a smoother way. The algorithm known as Perlin Noise does this. It generates pseudo-random numbers, where each number is close to the previous number.
Fun fact: Perlin Noise was created by Ken Perlin to be used in the movie Tron.
One of the best parts of this book is when Daniel Shiffman visually shows the difference between the random function and the noise (Perlin Noise) function.
He shows this through the vertex function, which creates vertices; you just need to pass an x (width) and a y (height) value.
In this case, the y (height) value is generated by random:
Vertices with heights generated from the random function (Source Code)
In this other case, y (height) is generated by noise:
Vertices with heights generated from the noise function (Source Code)
I don't know about you, but I found this amazing, the way the line is drawn organically, yet everything is generated randomly. It fascinated me.
Finally, the Walker with Perlin Noise:
A Simple Walker with Perlin Noise (Source Code)
The book continues explaining more topics, and I highly recommend reading it. It is completely free.
I will continue reading, and the next chapter will be about vectors. I am super excited and might comment on it in another post.